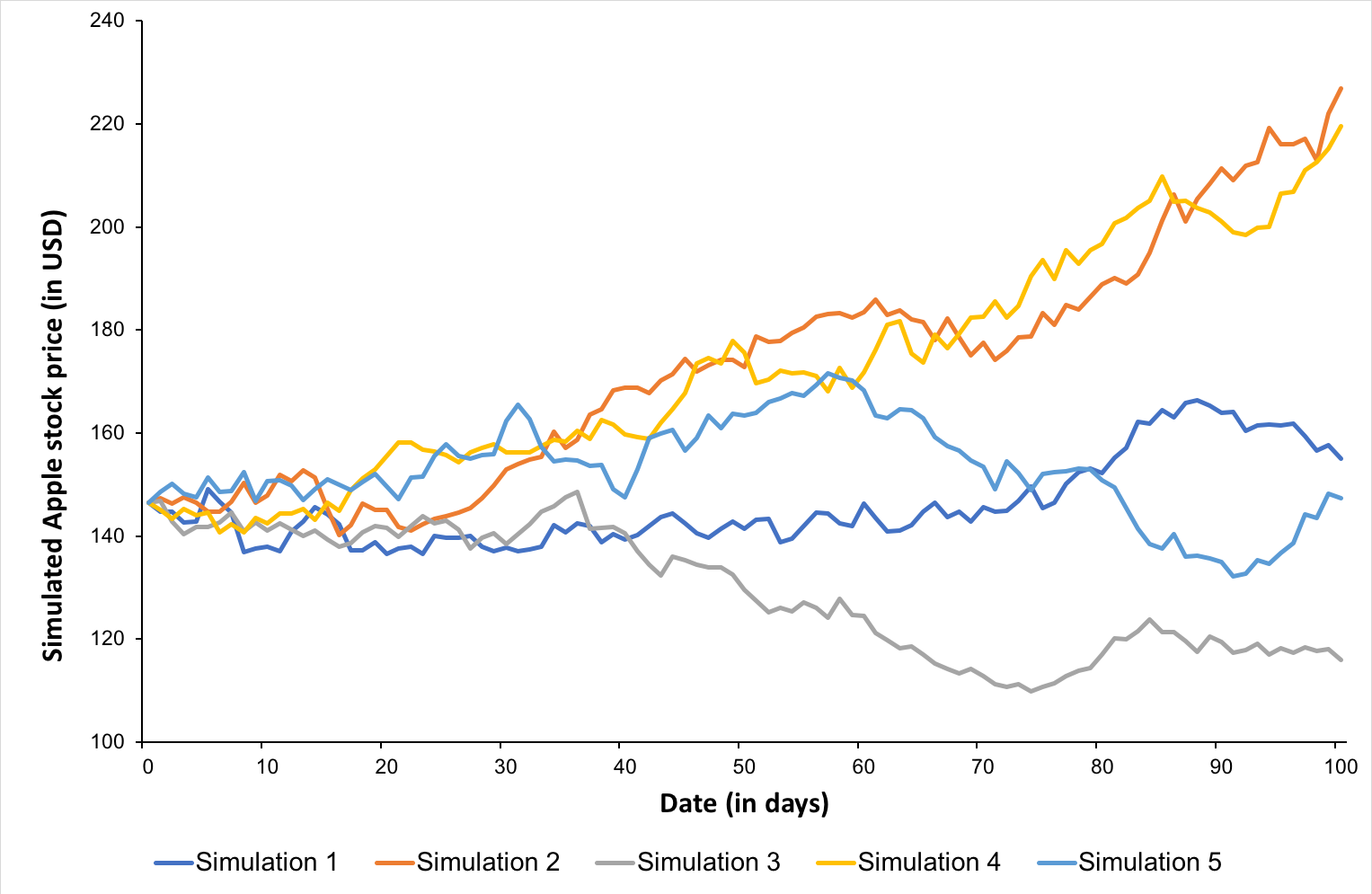
Monte Carlo Simulations in Finance
Advanced pricing of weather derivatives & convertible bonds using Python implementations with focus on practical calibration and risk management insights.
Introduction: Why Monte Carlo Methods Dominate Complex Valuation
In the realm of financial engineering, Monte Carlo (MC) simulations have emerged as the gold standard for pricing instruments where closed-form solutions fail. Unlike traditional models (Black-Scholes, binomial trees), MC methods excel at handling:
✔ Path-dependent payoffs (e.g., Asian options, barrier clauses)
✔ Multiple stochastic factors (equity prices, interest rates, weather variables)
✔ Early exercise features (convertible bonds, American options)
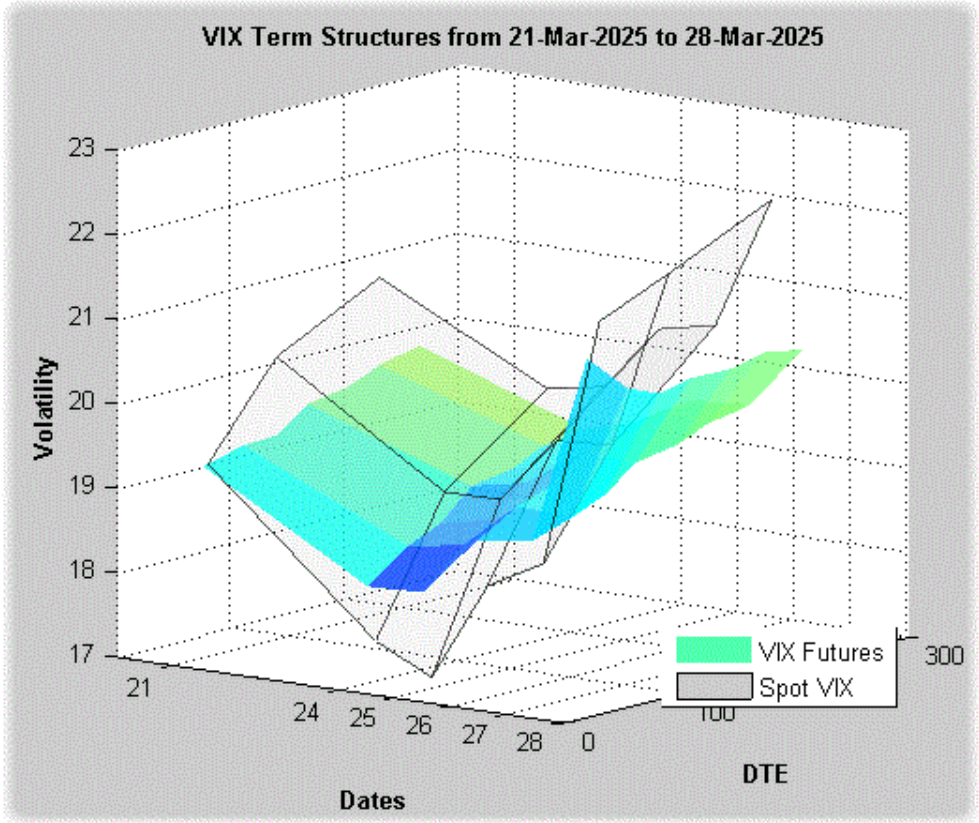
Figure 1: Monte Carlo simulation paths for temperature modeling
Weather Derivatives: Pricing Climate Uncertainty
Weather derivatives are OTC contracts tied to metrics like:
- Temperature (HDD/CDD indices)
- Precipitation (rainfall/swaps for agriculture)
- Wind speed (renewable energy hedging)
Key buyers: Utilities, insurers, agribusinesses hedging revenue volatility.
Modeling Temperature: Ornstein-Uhlenbeck Process
Temperature exhibits mean reversion—a core trait captured by the Ornstein-Uhlenbeck (OU) process:
dTₜ = θ(μ - Tₜ)dt + σdWₜ
Where:
- Tₜ = Temperature at time t
- μ = Long-term mean (e.g., 20°C for London)
- θ = Mean reversion speed (calibrated to historical data)
- σ = Volatility (higher in winter/summer)
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
def simulate_temperature(T0, mu, theta, sigma, days, n_sims):
"""
Simula trayectorias de temperatura usando el proceso de Ornstein-Uhlenbeck (OU).
"""
dt = 1 / 365 # Pasos diarios
paths = np.zeros((n_sims, days))
paths[:, 0] = T0
for t in range(1, days):
dW = np.random.normal(0, np.sqrt(dt), n_sims)
paths[:, t] = paths[:, t - 1] + theta * (mu - paths[:, t - 1]) * dt + sigma * dW
return paths
# Parámetros de temperatura
T0, mu, theta, sigma = 15, 20, 0.5, 2.5
n_sims = 500 # Más simulaciones
days = 365 # Un año
# Generar simulaciones
np.random.seed(42) # Reproducibilidad
temperature_paths = simulate_temperature(T0, mu, theta, sigma, days, n_sims)
# Calcular Heating Degree Days (HDDs)
HDDs = np.sum(np.maximum(18 - temperature_paths, 0), axis=1)
# Crear figura con múltiples gráficos
fig, axs = plt.subplots(2, 2, figsize=(12, 10))
# Gráfico 1: Trayectorias de temperatura
for i in range(20): # Solo 20 trayectorias para claridad
axs[0, 0].plot(temperature_paths[i], alpha=0.7)
axs[0, 0].axhline(mu, color='red', linestyle='--', label='Media a largo plazo (μ)')
axs[0, 0].set_title('Simulación Monte Carlo de Temperatura (OU Process)')
axs[0, 0].set_xlabel('Días')
axs[0, 0].set_ylabel('Temperatura (°C)')
axs[0, 0].legend()
axs[0, 0].grid(True)
# Gráfico 2: Histograma de temperaturas finales
sns.histplot(temperature_paths[:, -1], bins=30, kde=True, ax=axs[0, 1])
axs[0, 1].set_title('Distribución de Temperaturas al Final del Año')
axs[0, 1].set_xlabel('Temperatura Final (°C)')
axs[0, 1].set_ylabel('Frecuencia')
# Gráfico 3: Histogramas de Heating Degree Days (HDDs)
sns.histplot(HDDs, bins=30, kde=True, ax=axs[1, 0])
axs[1, 0].set_title('Distribución de Heating Degree Days (HDDs)')
axs[1, 0].set_xlabel('HDD')
axs[1, 0].set_ylabel('Frecuencia')
# Gráfico 4: Boxplot de HDDs
sns.boxplot(x=HDDs, ax=axs[1, 1])
axs[1, 1].set_title('Boxplot de HDDs')
axs[1, 1].set_xlabel('Heating Degree Days')
# Ajustar disposición y mostrar
plt.tight_layout()
plt.show()
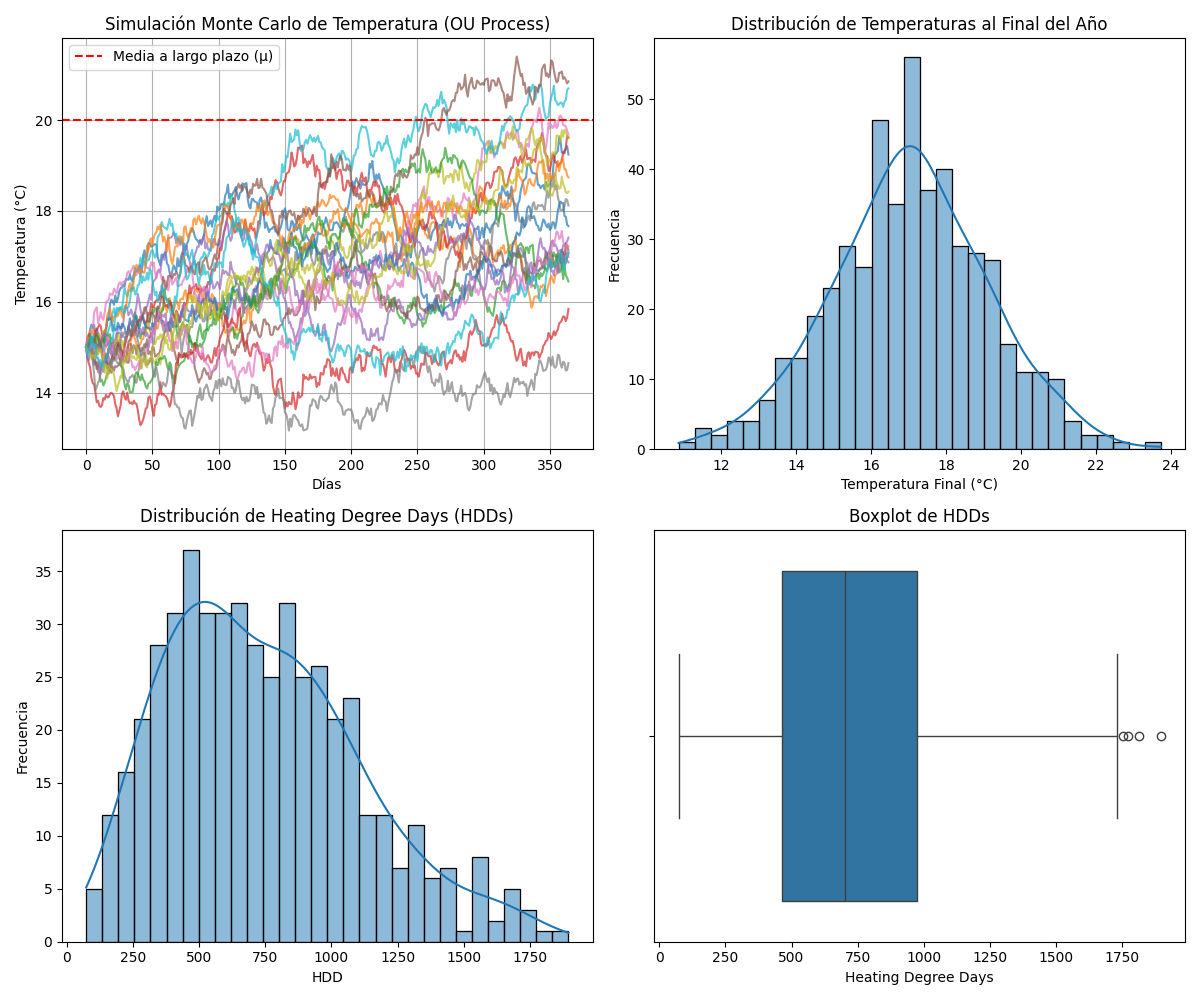
Convertible Bonds: Valuing Hybrid Securities
Convertible bonds combine debt features (coupon payments, principal repayment) with an embedded equity option allowing conversion into stock. Pricing is complex due to:
- Stock price evolution (modeled via geometric Brownian motion)
- Interest rate dynamics (e.g., Vasicek or CIR models)
- Optimal conversion strategy (American-style option feature)
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
def simulate_gbm(S0, mu, sigma, T, dt, N):
"""
Simula trayectorias de precios de acciones usando Movimiento Browniano Geométrico (GBM).
"""
np.random.seed(42)
paths = np.zeros((N, int(T / dt)))
paths[:, 0] = S0
for t in range(1, paths.shape[1]):
dW = np.random.normal(0, np.sqrt(dt), N)
paths[:, t] = paths[:, t - 1] * np.exp((mu - 0.5 * sigma**2) * dt + sigma * dW)
return paths
def simulate_vasicek(r0, theta, mu, sigma, T, dt, N):
"""
Simula trayectorias de tasas de interés usando el modelo de Vasicek.
"""
np.random.seed(42)
paths = np.zeros((N, int(T / dt)))
paths[:, 0] = r0
for t in range(1, paths.shape[1]):
dW = np.random.normal(0, np.sqrt(dt), N)
paths[:, t] = paths[:, t - 1] + theta * (mu - paths[:, t - 1]) * dt + sigma * dW
return paths
# Parámetros de simulación
S0, mu_stock, sigma_stock, T, dt, N = 100, 0.05, 0.2, 5, 1/252, 500
r0, theta, mu_rate, sigma_rate = 0.03, 0.1, 0.05, 0.02
# Generar simulaciones
stock_paths = simulate_gbm(S0, mu_stock, sigma_stock, T, dt, N)
rate_paths = simulate_vasicek(r0, theta, mu_rate, sigma_rate, T, dt, N)
# Crear figura con múltiples gráficos
fig, axs = plt.subplots(2, 2, figsize=(12, 10))
# Gráfico 1: Simulación de precios de acciones
for i in range(20):
axs[0, 0].plot(stock_paths[i], alpha=0.7)
axs[0, 0].set_title('Simulación Monte Carlo de Precios de Acciones (GBM)')
axs[0, 0].set_xlabel('Días')
axs[0, 0].set_ylabel('Precio de Acción')
axs[0, 0].grid(True)
# Gráfico 2: Distribución de precios finales de acciones
sns.histplot(stock_paths[:, -1], bins=30, kde=True, ax=axs[0, 1])
axs[0, 1].set_title('Distribución de Precios Finales de Acciones')
axs[0, 1].set_xlabel('Precio Final de Acción')
axs[0, 1].set_ylabel('Frecuencia')
# Gráfico 3: Simulación de tasas de interés
for i in range(20):
axs[1, 0].plot(rate_paths[i], alpha=0.7)
axs[1, 0].set_title('Simulación Monte Carlo de Tasas de Interés (Vasicek)')
axs[1, 0].set_xlabel('Días')
axs[1, 0].set_ylabel('Tasa de Interés')
axs[1, 0].grid(True)
# Gráfico 4: Distribución de tasas de interés finales
sns.histplot(rate_paths[:, -1], bins=30, kde=True, ax=axs[1, 1])
axs[1, 1].set_title('Distribución de Tasas de Interés Finales')
axs[1, 1].set_xlabel('Tasa de Interés Final')
axs[1, 1].set_ylabel('Frecuencia')
# Ajustar disposición y mostrar
plt.tight_layout()
plt.show()
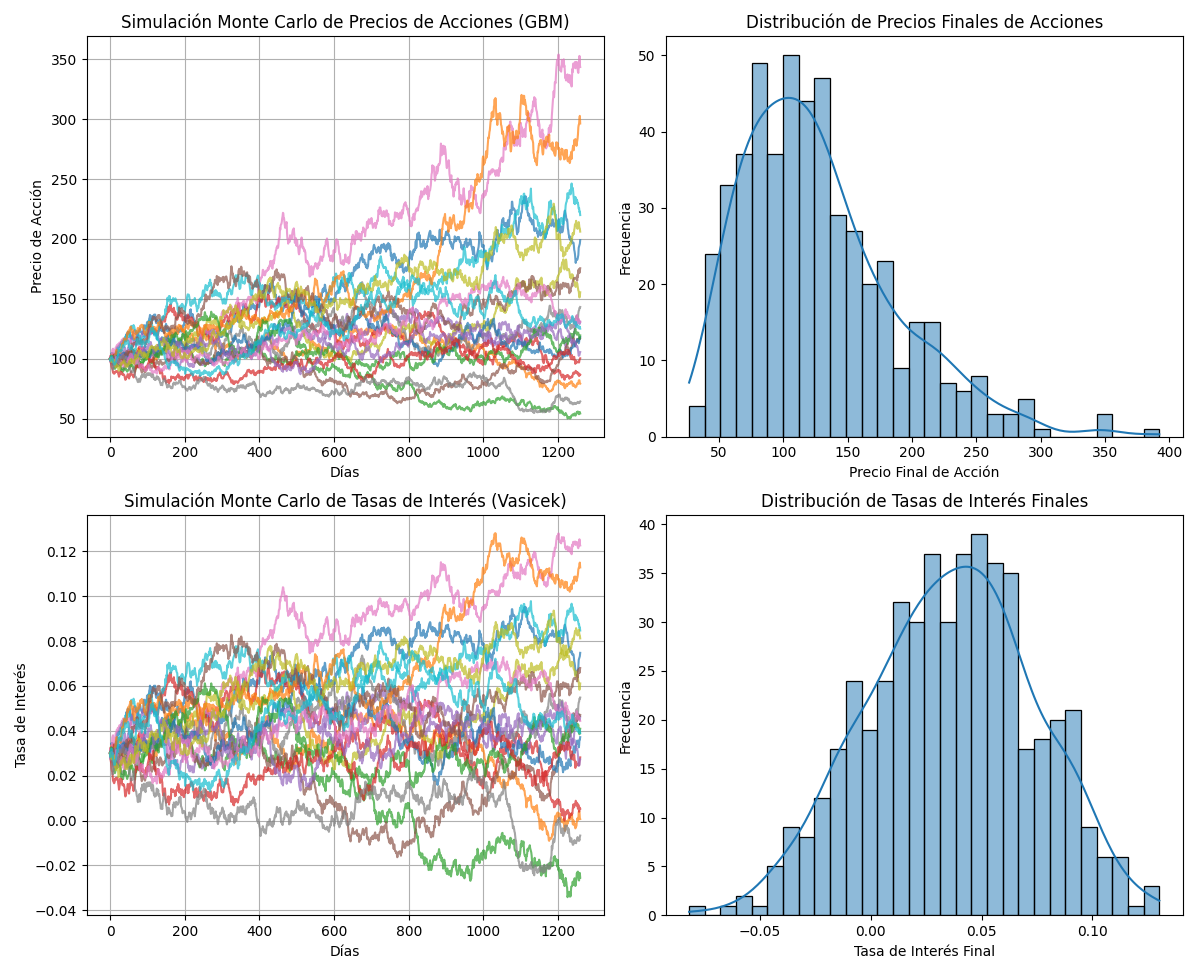
Key Takeaways
1. Monte Carlo simulations provide robust pricing for complex, path-dependent securities.
2. Weather derivatives benefit from stochastic temperature modeling (OU process).
3. Convertible bonds require joint simulation of stock prices and interest rates.
4. Python implementation allows flexible scenario analysis and risk assessment.
Monte Carlo methods are indispensable for modern financial engineering, enabling precise valuation of structured products in uncertain environments. By integrating market data and advanced calibration techniques, analysts can derive actionable insights for investment and risk management.